일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- innerText
- javascript
- Review
- HTML
- js
- node
- CSS
- es6
- 앱 시장 조사
- textContent
- modal
- snyk
- Event
- expo
- innerHTML
- Dom
- eas
- react
- ES5
- yet
- Empty
- 빌드 전 점검
- 앱 마케팅 전략
- a11y
- eas build
- 커스텀 테마
- UI
- node.js
- addEventListener
- nodeValue
- Today
- Total
the murmurous sea
JS ES6: Promise 본문
1. object
2. represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
3. allows you to associate handlers with an asynchronous action's eventual success value or failure reason.
4. guaranteed to be asynchronous
: Therefore, an action for an already "settled" promise will occur only after the stack has cleared and a clock-tick has passed.
: The effect is much like that of setTimeout(action,10).
: example code ▼
const promiseA = new Promise( (resolutionFunc,rejectionFunc) => { resolutionFunc(777); }); // At this point, "promiseA" is already settled. promiseA.then( (val) => console.log("asynchronous logging has val:",val) ); console.log("immediate logging"); // produces output in this order: // immediate logging // asynchronous logging has val: 777
5. This lets asynchronous methods return values like synchronous methods
: instead of immediately returning the final value, the asynchronous method returns a promise to supply the value at some point in the future.
6. A Promise is in one of these states:
pending | initial state, neither fulfilled nor rejected. |
fulfilled | meaning that the operation completed successfully. |
rejected | meaning that the operation failed. |
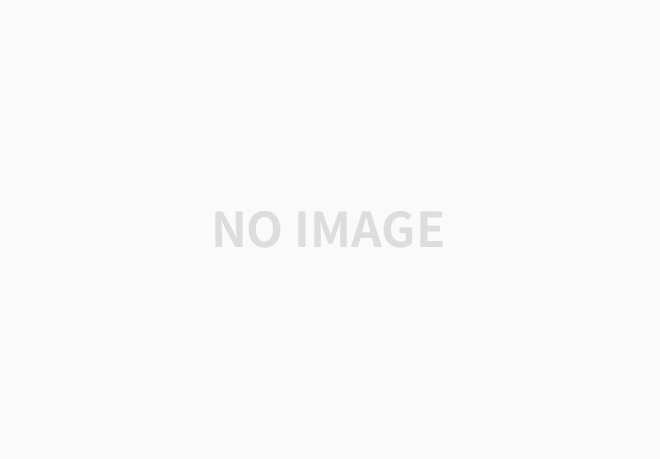
7. As the Promise.prototype.then() and Promise.prototype.catch() methods return promises, they can be chained.
8. A promise is said to be settled if it is either fulfilled or rejected, but not pending.
: You will also hear the term resolved used with promises — this means that the promise is settled or “locked in” to match the state of another promise.
[Constructor]
: Creates a new Promise object.
: The constructor is primarily used to wrap functions that do not already support promises.
Promise()
Not to be confused
: with several other languages have mechanisms for lazy evaluation and deferring a computation, which they also call "promises", e.g. Scheme.
Promises in JavaScript
: represent processes that are already happening, which can be chained with callback functions.
: If you are looking to lazily evaluate an expression, consider the arrow function with no arguments: f = () => expression to create the lazily-evaluated expression, and f() to evaluate.
Chained Promises
1. The methods are used to associate further action with a promise that becomes settled.
: promise.then(), promise.catch(), and promise.finally()
2. These methods also return a newly generated promise object,
3. which can optionally be used for chaining.
const myPromise = (new Promise(myExecutorFunc)) .then(handleFulfilledA,handleRejectedA) .then(handleFulfilledB,handleRejectedB) .then(handleFulfilledC,handleRejectedC); // or, perhaps better ... const myPromise = (new Promise(myExecutorFunc)) .then(handleFulfilledA) .then(handleFulfilledB) .then(handleFulfilledC) .catch(handleRejectedAny);
promise.then() | ||
promise.catch() | ||
promise.finally() |
4. It is simpler to leave out error handling until a final .catch() statement
: in the absence of an immediate need.
: Handling a rejected promise too early has consequences further down the promise chain.
- Sometimes there is no choice, because an error must be handled immediately.
5. When a .then() lacks the appropriate function, processing simply continues to the next link of the chain.
: Therefore, a chain can safely omit every handleRejection until the final .catch().
: Similarly, .catch() is really just a .then() without a slot for handleFulfilled.
6. The termination condition of these functions determines the "settled" state of the next promise in the chain.
: Any termination other than a throw creates a "resolved" state, while terminating with a throw creates a "rejected" state.
handleFulfilled(value) { /*...*/; return nextValue; } handleRejection(reason) { /*...*/; throw nextReason; } handleRejection(reason) { /*...*/; return nextValue; }
7. The promises of a chain are nested.
: When a nextValue is a promise, the effect is a dynamic replacement.
: The return causes a promise to be popped, but the nextValue promise is pushed into its place.
[Static methods]
returns | ||
Promise.all(iterable) | Wait for all promises to be resolved, or for any to be rejected. | resolved : with an aggregating array of the values from the resolved promises (in the same order as defined in the iterable of multiple promises.) rejected : with the reason from the first promise in the iterable that was rejected. |
Promise.allSettled(iterable) | Wait until all promises have settled (each may resolve or reject). | a promise that resolves after all of the given promises have either resolved or rejected, with an array of objects that each describe the outcome of each promise. |
Promise.race(iterable) | Wait until any of the promises is resolved or rejected. | it is resolved(or rejected) with the value(or the reason) of the first promise in the iterable that resolved/rejected. |
Promise.reject(reason) | Returns a new Promise object that is rejected with the given reason. | a new Promise object that is rejected with the given reason. |
Promise.resolve(value) | - Returns a new Promise object that is resolved with the given value. - If the value has a then method, the returned promise will "follow" that. - Generally, use this method instead and work with the return value as a promise if you don't know if a value is a promise or not. |
a new Promise object that is resolved with the given value. |
[Instance methods]
Appends | returns | |
Promise.prototype.catch() | A rejection handler callback to the promise | a new promise resolving to the return value of the callback if it is called |
a new promise resolving to its original fulfillment value if the promise is instead fulfilled. | ||
Promise.prototype.then() | Fulfillment and rejection handlers to the promise | a new promise resolving to the return value of the called handler |
a new promise resolving to its original settled value if the promise was not handled | ||
Promise.prototype.finally() | A handler to the promise. The handler is called when the promise is settled, whether fulfilled or rejected. |
a new promise that is resolved when the original promise is resolved |
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise
Promise
The Promise object represents the eventual completion (or failure) of an asynchronous operation, and its resulting value.
developer.mozilla.org
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Using_promises
Using Promises
A Promise is an object representing the eventual completion or failure of an asynchronous operation. Since most people are consumers of already-created promises, this guide will explain consumption of returned promises before explaining how to create them.
developer.mozilla.org
↑didn't study yet
'#dev > 개념정리' 카테고리의 다른 글
SPA(Single Page Application) (0) | 2020.05.21 |
---|---|
JS: Promise.prototype.then() - incompleted (0) | 2020.05.21 |
JS ES6: symbol (0) | 2020.05.15 |
생성자 함수 (empty) (0) | 2020.05.14 |
JS: split() vs. join() (0) | 2020.05.14 |